STORIES
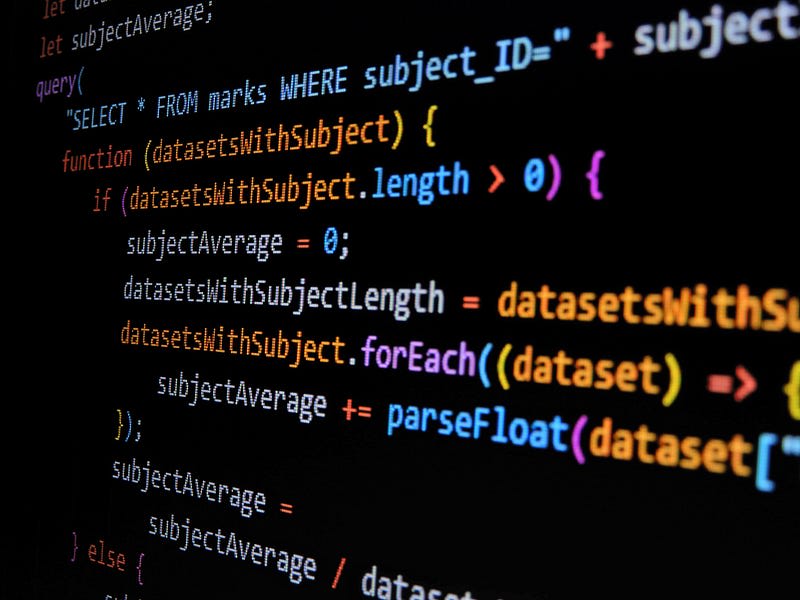
My name is Daan, I’m a Technical Architect at Triple and in this blog, I will share my experiences with Node.js and tell you what to pay attention to if you are already familiar with PHP. Node.js is a powerful JavaScript runtime environment that eliminates the need to work with different programming languages for server-side and client-side scripts.
According to Wikipedia, PHP was born on 8 June 1995, which is 27 years ago at the time of writing this blog. I started writing PHP scripts around the age of 16, in the year 2000, when PHP version 4 had already been launched.
At that time, technically there were only two options: PHP and ASP.net. PHP was more Linux-oriented and ASP.net was (and still is) only available for Windows. Perl was an option as well, but it felt more like a scripting language than a language for building a complete web application.
Fast-forward to now. PHP still plays an important role. According to kinsta.com, approximately 80% of all websites still rely on PHP to some extent. The number of available techniques has increased significantly since that time:
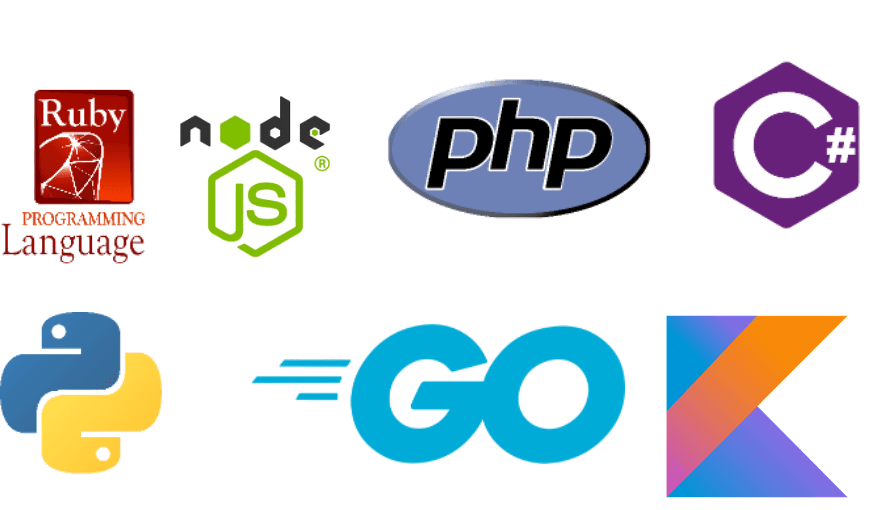
Why Node.js?
I started developing in Node.js (alongside PHP) about six years ago, mainly in side projects. I had already become familiar with JavaScript while building web applications for Triple. Previously, I had also been investigating which techniques were generally used in the front-end. As a result, I had already gained some experience with Preact and server-side rendering, which is what Node.js does. The toolchain for building those front-end applications is also found in Node. Another important consideration to continue with Node.js is that those applications were easier to deploy on my Raspberry Pi at home. With php-fpm and nginx, it’s harder to have different PHP versions running in parallel on the same server.
What you need to know about Node.js as a PHP developer
1 — Pay attention to the handling of processes and requests
Node.js operates in a different way than PHP. With PHP, in most cases a request is received by nginx or Apache and passed on to php-fpm. A Node application is a process that you start yourself. In the code you configure a web server (e.g. Express) that listens on a TCP port. Besides, you configure the routes directly on that web server.
In PHP, the php-fpm process is responsible for calling the PHP interpreter. In Node.js, the request is handled directly within the application itself. As a result, in Node.js you should be very careful not to manipulate anything outside the request. Unlike PHP, where the entire script ends when the request is completed, Node.js keeps the process running.
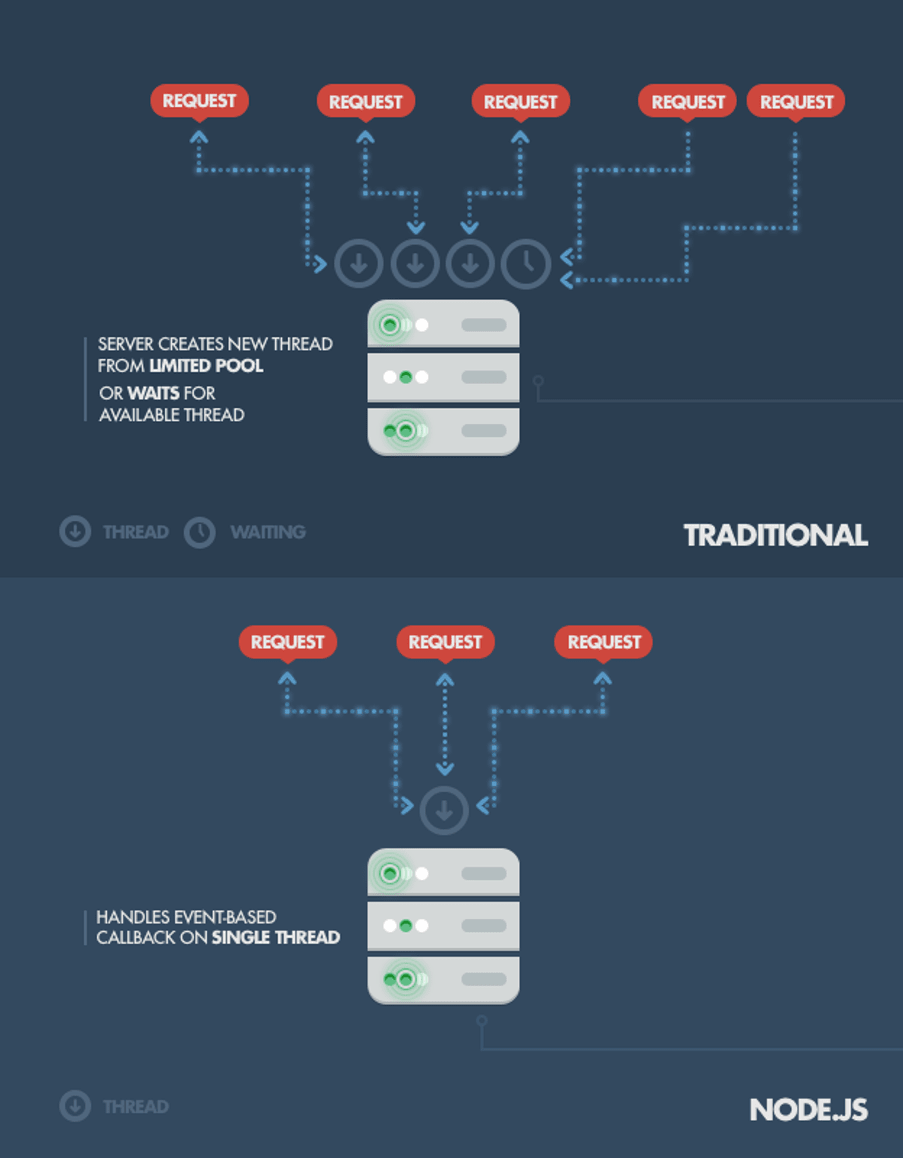
In the example below, the value of counter keeps increasing between requests. This would not be possible in PHP.
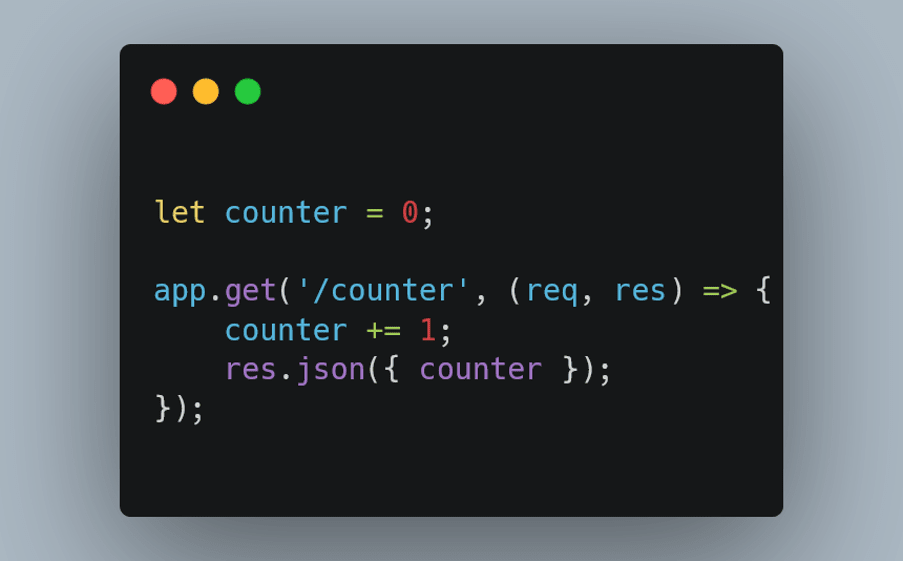
2 — Choose the right package manager
PHP uses composer, combined with packagist.org. In Node, npmjs.com is the equivalent of packagist.org. There are various package managers available, the two most popular are:
1. Npm, the default package manager from Node.
2. Yarn, a package manager that originated in Facebook.
It would be going too far to go into the differences, advantages and disadvantages of the two now. As a novice Node.js developer, the choice for npm is the easiest, because it is already available by default.
The dependencies and packages to be installed are defined in a package.json file in the project root. After installing packages, a package-lock.json file is created, which is the equivalent of the composer.lock file.
After running an npm install command, all dependencies are downloaded to the node_modules directory. From there, the dependencies are incorporated into the project as required.
3 — Use TypeScript from the start
“TypeScript is a programming language developed and maintained by Microsoft. It is a strict syntactical superset of JavaScript and adds optional static typing to the language. It is designed for the development of large applications and transpiles to JavaScript.[4] As it is a superset of JavaScript, existing JavaScript programs are also valid TypeScript programs.” Source: Wikipedia
If you choose to develop with Node.js, my advice is to start directly with TypeScript. The big advantage is that during development, you can already give types to variables, arguments and so on. This way, you are already informed of any problems in your IDE, where with normal JavaScript this only happens during code execution.
An example of the same application, in JavaScript and in TypeScript.
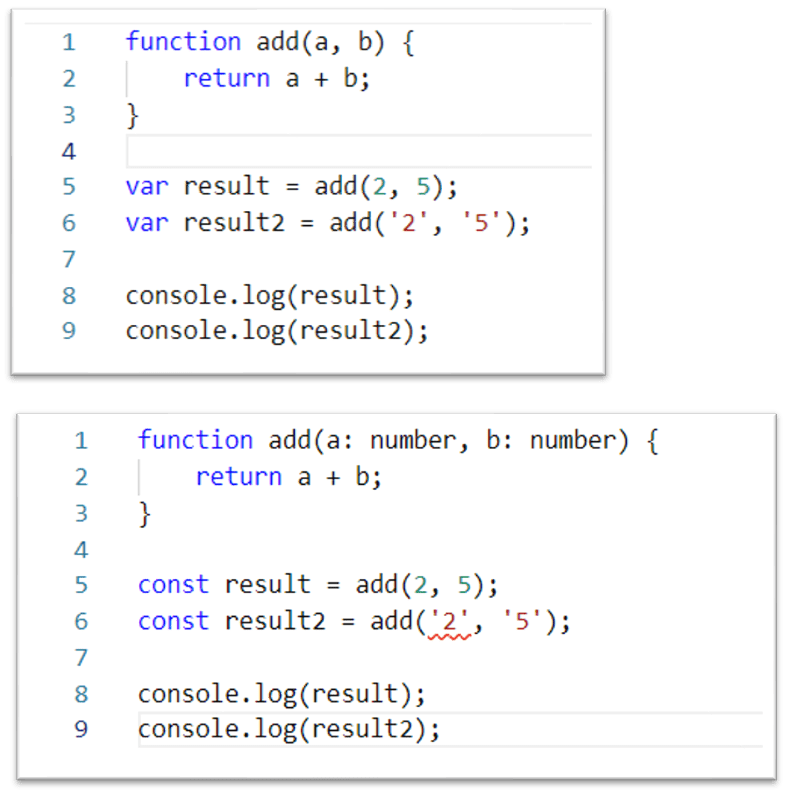
Via a build step, the TypeScript code is converted into JavaScript code. The typings and such are therefore only available during development. For the output, the TypeScript code is transpiled to JavaScript and will be interpreted accordingly. It is therefore essential to validate the structure of external data that the application receives, instead of just indicating that the data has a certain structure. If you don’t, you will not receive a warning during development, but problems will occur during execution.
Below you see an example of the transpilation of TypeScript to JavaScript. As JavaScript cannot contain the typings, you can indicate in the build step of TypeScript that you would like the type declarations to be generated in a separate file. This is mostly used with external modules, because you can still indicate what the typings are of the imported JavaScript code.
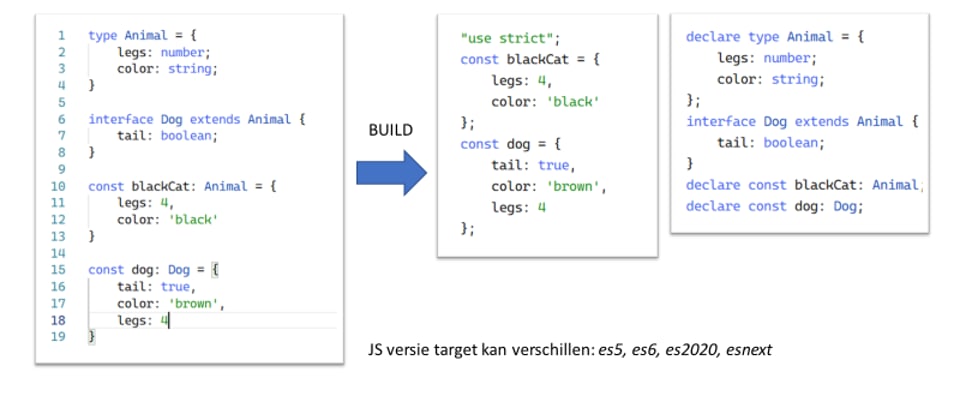
4 — Pay attention to the import and export of modules
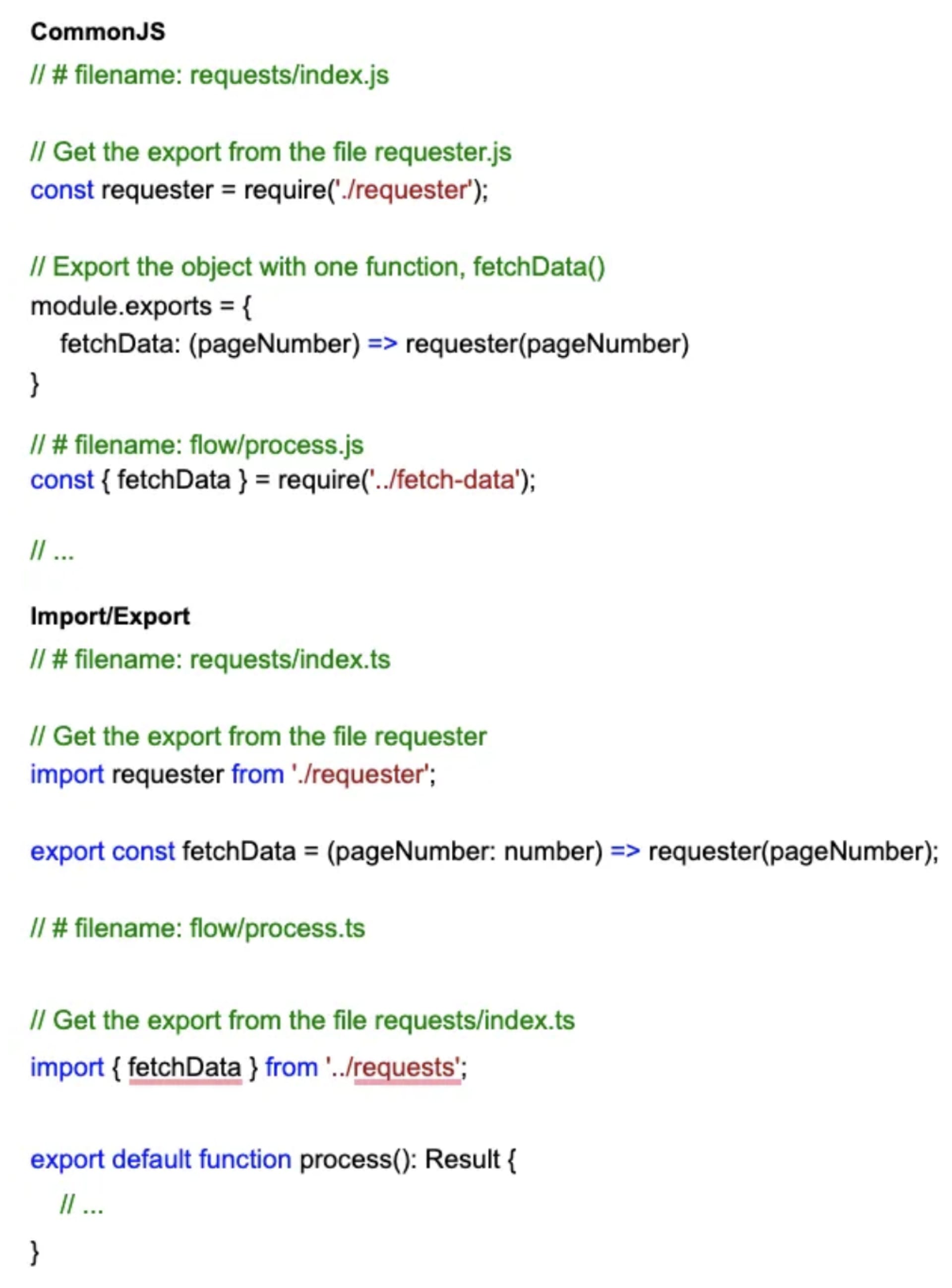
5 — View the information on versioning and Long Term Support
For Node.js there is a clear strategy for the Long Term Support (LTS) of versions. This information can be found on the website.
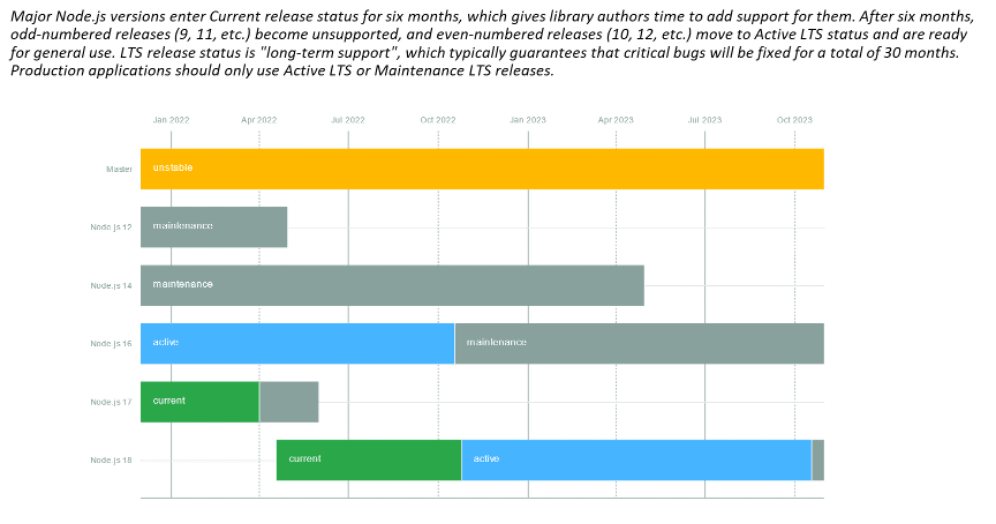
6 — Learn to work with classes, functions and anonymous functions
See below for some examples of notation of functions in classes in JavaScript.
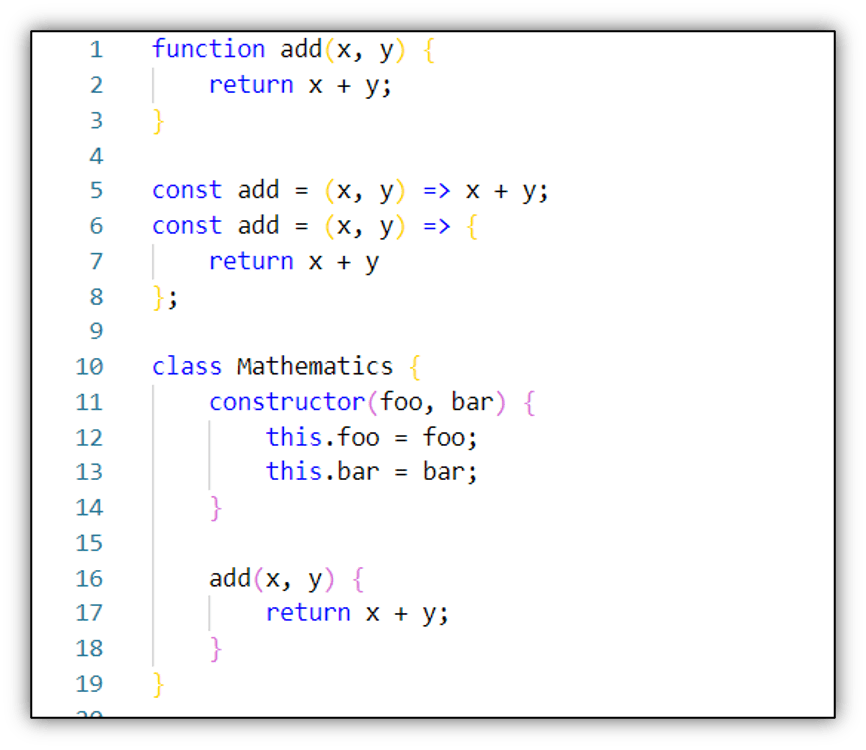
7 — Use promises / async / await
“The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value.” — https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise
By using promises, you can allow program execution to continue while waiting for a particular action (such as querying a database) to complete. As Node.js is single-threaded, synchronous actions will block the application’s output for each HTTP request until the operation completes. Promises are the solution to this. Until recently, we worked with callbacks, where the result of the operation was returned via a function call.
Below you can see an example of a function that returns a promise and how the calling function handles this. The function in then will be called with the result of the promise.
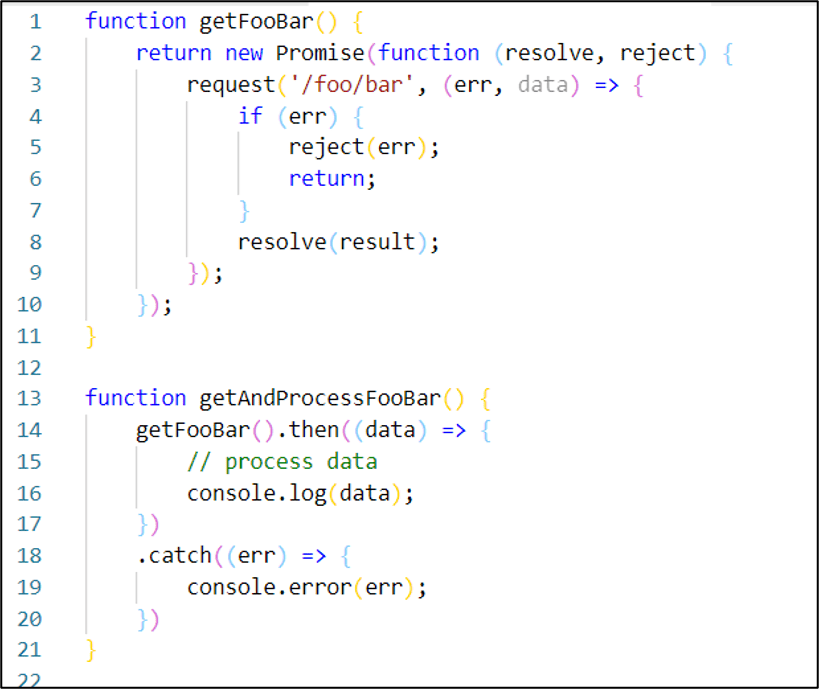
The mechanisms Async/await make working with promises easier. If a function uses await, it must be marked as asynchronous with the keyword async. Within the function, you can then type await fooBar(). This translates to a fooBar().then() in the interpretation, but you do not have to type it explicitly. The example above can therefore be written in a much more readable way:
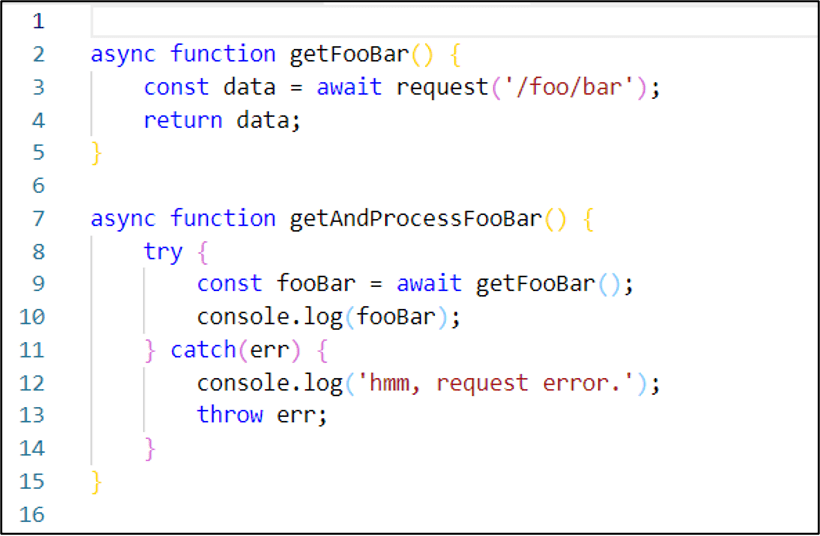
8 — Note the absence of autoloading / namespaces
The concepts of autoloading and namespacing do not exist within Node.js. Namespacing is achieved by already specifying the location in the import/require statement.
Futhermore, Dependency Injection, a well-known design pattern with many advantages, is not used very often. This is the pattern I miss the most in the JavaScript ecosystem.
9 — Choose the HTTP framework that suits you
Within a Node.js process, as a developer you are responsible for the handling of HTTP requests. Node.js offers a low-level module for this: http.
In fact, the HTTP module is never built on directly. Rather, a framework is used which works on top of the HTTP module.
Express is a framework that works directly on top of http. Additionally, there are various frameworks that are built on top of Express: Koa, Hapi and fastify, among others.
As with PHP, the choice of framework is a personal one. It also depends on what your requirements are and what you are used to. My personal preference is the use of Express, the main reason being that I have extensive experience with it. The biggest disadvantage of Express is that it does not automatically handle async request handlers properly. Express 5 should solve this, but it is still in beta at the moment. In this article you can read more about this subject.
10 — Make use of tooling
The JavaScript ecosystem is famous (or notorious) for its many frameworks and tools that solve similar problems. In order to keep seeing the woods for the trees, I will highlight some of them.
Prettier On Prettier, the makers call it an opiniated code formatter. It is comparable to tools like phpcs or php-cs-fixer. By running Prettier in the project, a number of coding formatting standards (tabs vs spaces, newlines and so on) are applied to each file. With an integration into your applied IDE, you can even have the formatting done while saving the file.
Nodemon Developing in Node.js works differently to developing in PHP. In PHP, refreshing the page is enough to see code changes, in Node.js the application must be restarted. Nodemon is a simple tool which monitors the file system for changes in .js or .ts files. If changes are detected, the process is restarted so that the changes are immediately visible.
Eslint “ESLint is a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code.” This is a widely used tool within JavaScript. Whereas Prettier specializes in formatting, Eslint is a linting tool that also warns of content ‘issues’ with the code. It is comparable to phpstan.
Its application is simple: you create a set of rules (and possibly use a standard definition, such as airbnb or standard, as a basis) and your code is validated against that set of rules. What exactly the rules entail can vary from case to case. For example, it may concern the order of your imports, or whether you use a short-hand note for an if statement.
Jest The (unit) testing framework that is most popular at the moment is Jest. With unit testing, code snapshots and mocking, it is a tool with which you can easily set up all kinds of automatic tests.
More information?
In this blog, I have given ten tips for PHP developers who are about to start writing applications in Node.js. Do you disagree with me or do you have any questions? You can reach me at d.zonneveld@wearetriple.com. Looking for a new job or want to learn more about Triple? Check out our website.
Explore the Triple Universe
Learn more about Triple, our culture or check out our vacancies.